Creating folders in Java is an essential skill for developers, especially for those who work with file management and organization. The ability to create directories programmatically allows for efficient storage, retrieval, and categorization of files. Whether you are developing a desktop application, a web service, or a simple utility program, understanding how to create folders in Java can enhance your application's functionality and usability. In this article, we will delve into the various methods and best practices for creating folders using Java. We will explore the built-in libraries that Java offers, as well as provide code snippets and practical examples to illustrate the process.
Folder creation is a common requirement in many software projects, ranging from saving user-generated content to organizing resources for applications. As we navigate through this guide, you will learn about the significance of managing file systems effectively. Additionally, we will discuss error handling, permissions, and platform-specific considerations that come into play when creating folders in Java.
By the end of this article, you will be equipped with the knowledge and skills needed to implement folder creation in your Java applications. Whether you are a novice or an experienced developer, this guide aims to sharpen your understanding of file management in Java, ensuring you can handle directories with confidence and ease.
What is the Importance of Creating Folders in Java?
Creating folders in Java is crucial for several reasons. First, it helps in organizing files systematically, which is essential for both developers and end-users. Well-structured directories make it easier to locate and manage files, leading to improved efficiency. Second, folder creation allows applications to handle user data securely, as sensitive information can be stored in separate directories. Lastly, it provides a way to manage resources dynamically, adapting to user needs or application requirements.
How Can You Create a Folder in Java?
In Java, creating a folder can be achieved using various methods. The most common approach is to utilize the java.nio.file
package, which offers a modern and flexible way to handle file operations. Below is a simple example of how to create a folder using Java:
import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class CreateFolderExample { public static void main(String[] args) { Path path = Paths.get("exampleFolder"); try { Files.createDirectory(path); System.out.println("Folder created successfully!"); } catch (IOException e) { System.out.println("An error occurred: " + e.getMessage()); } } }
What Libraries are Available for Folder Creation in Java?
Java provides several libraries for handling file and folder creation, including:
- java.io: The traditional file handling library that offers basic file and directory operations.
- java.nio.file: A more modern library introduced in Java 7, providing advanced file I/O capabilities.
- Apache Commons IO: A third-party library that simplifies file and folder operations significantly.
What are the Best Practices for Creating Folders in Java?
When creating folders in Java, consider the following best practices:
- Always check if the folder already exists to avoid exceptions.
- Implement proper error handling to manage exceptions gracefully.
- Use relative paths for better portability across different environments.
- Consider using the
java.nio.file.Files
class for more advanced features.
How to Handle Exceptions When Creating Folders?
Exception handling is vital when creating folders in Java. Common exceptions to handle include:
- FileAlreadyExistsException: Thrown if the directory already exists.
- SecurityException: Thrown if the application lacks the necessary permissions.
- IOException: A general error that can occur during folder creation.
Here’s an example of how to handle exceptions while creating a folder:
import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.FileAlreadyExistsException; import java.io.IOException; public class CreateFolderWithExceptionHandling { public static void main(String[] args) { Path path = Paths.get("newFolder"); try { Files.createDirectory(path); System.out.println("Folder created successfully!"); } catch (FileAlreadyExistsException e) { System.out.println("Folder already exists: " + e.getMessage()); } catch (SecurityException e) { System.out.println("Permission denied: " + e.getMessage()); } catch (IOException e) { System.out.println("An unexpected error occurred: " + e.getMessage()); } } }
What Are the Platform-Specific Considerations for Creating Folders in Java?
When working with folder creation in Java, developers must consider platform-specific file system behaviors. For instance:
- File path separators differ between operating systems (e.g., "/" for UNIX-like systems and "\\" for Windows).
- Permission settings may vary, affecting the ability to create directories.
- Special characters in folder names may have different implications based on the OS.
What Are the Use Cases for Creating Folders in Java?
Creating folders in Java can be applied in various scenarios, including:
- File upload handling in web applications.
- Data storage for applications that require user-generated content.
- Organizing resources for game development.
- Managing configuration files and logs for applications.
Conclusion: How to Effectively Implement Java Create Folder?
In conclusion, mastering the process of creating folders in Java is a fundamental skill that enhances your file management capabilities. By leveraging the appropriate libraries and adhering to best practices, you can create a robust solution for handling directories in your applications. Practice the examples provided and implement the discussed techniques to ensure that your Java applications can manage folders efficiently and effectively.
You Might Also Like
Finding The Perfect Middle Names For AlexandraIlluminate Your Space: The Ultimate Guide To Replacement Lamp Light Sockets
Experience Culinary Delight At Vit Vit Restaurant
Unleashing Innovation: The Volcanic Arms Company Revolution
Understanding Upper Molar RCT: A Comprehensive Guide
Article Recommendations
- Profile Of Kari Hillsman A Multifaceted Personality With A Rich Legacy
- The Intriguing Age Of Olivia Benson A Deep Dive Into Her Timeless Character
- Michael Schoeffling Furniture Website A New Chapter In Craftsmanship

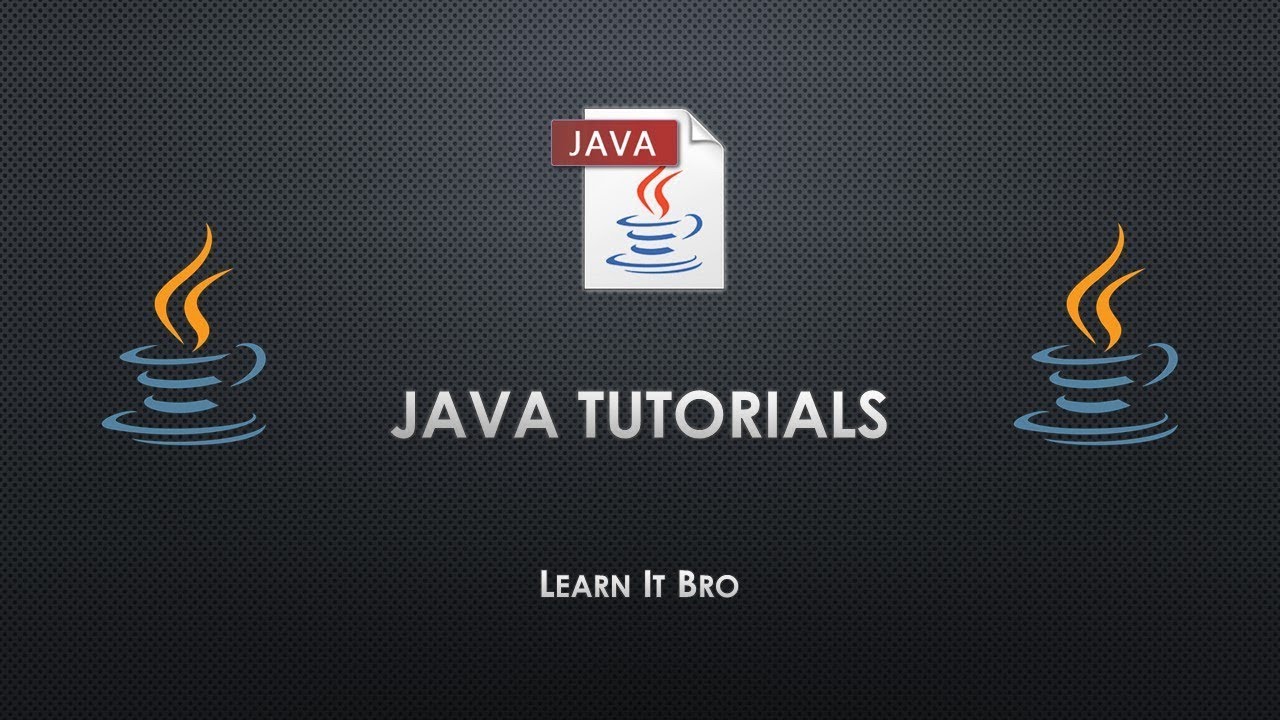
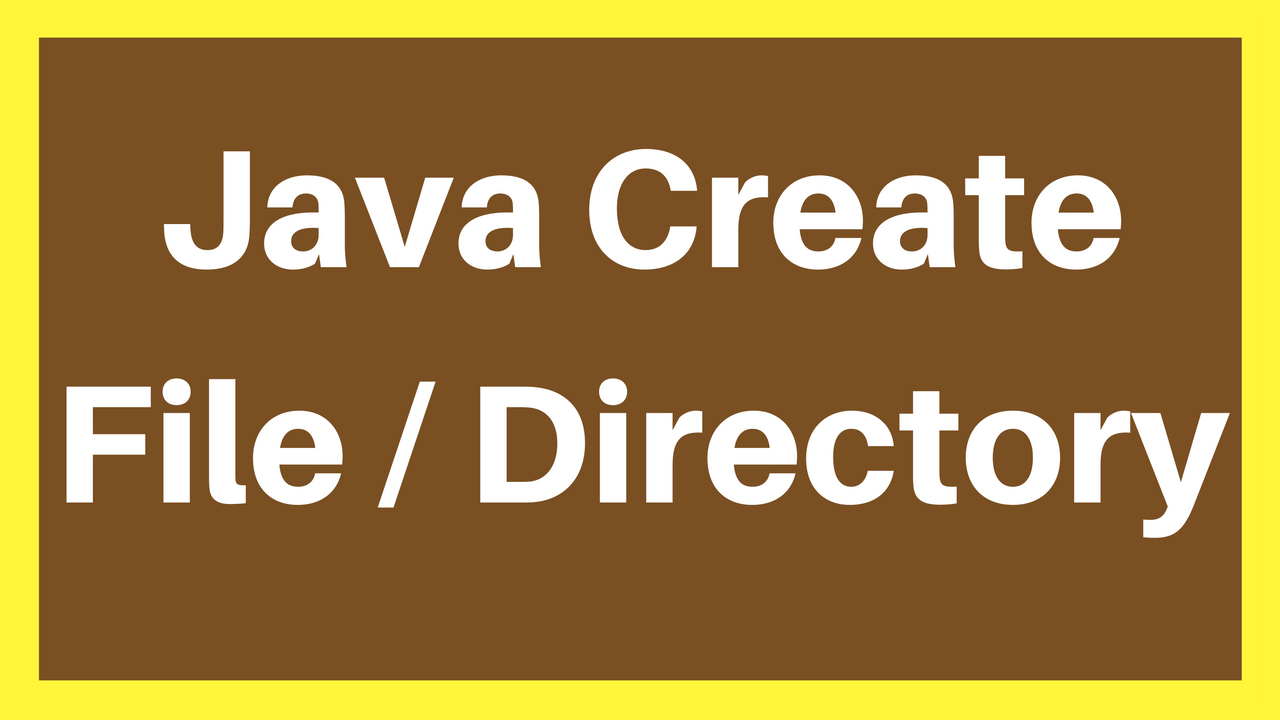